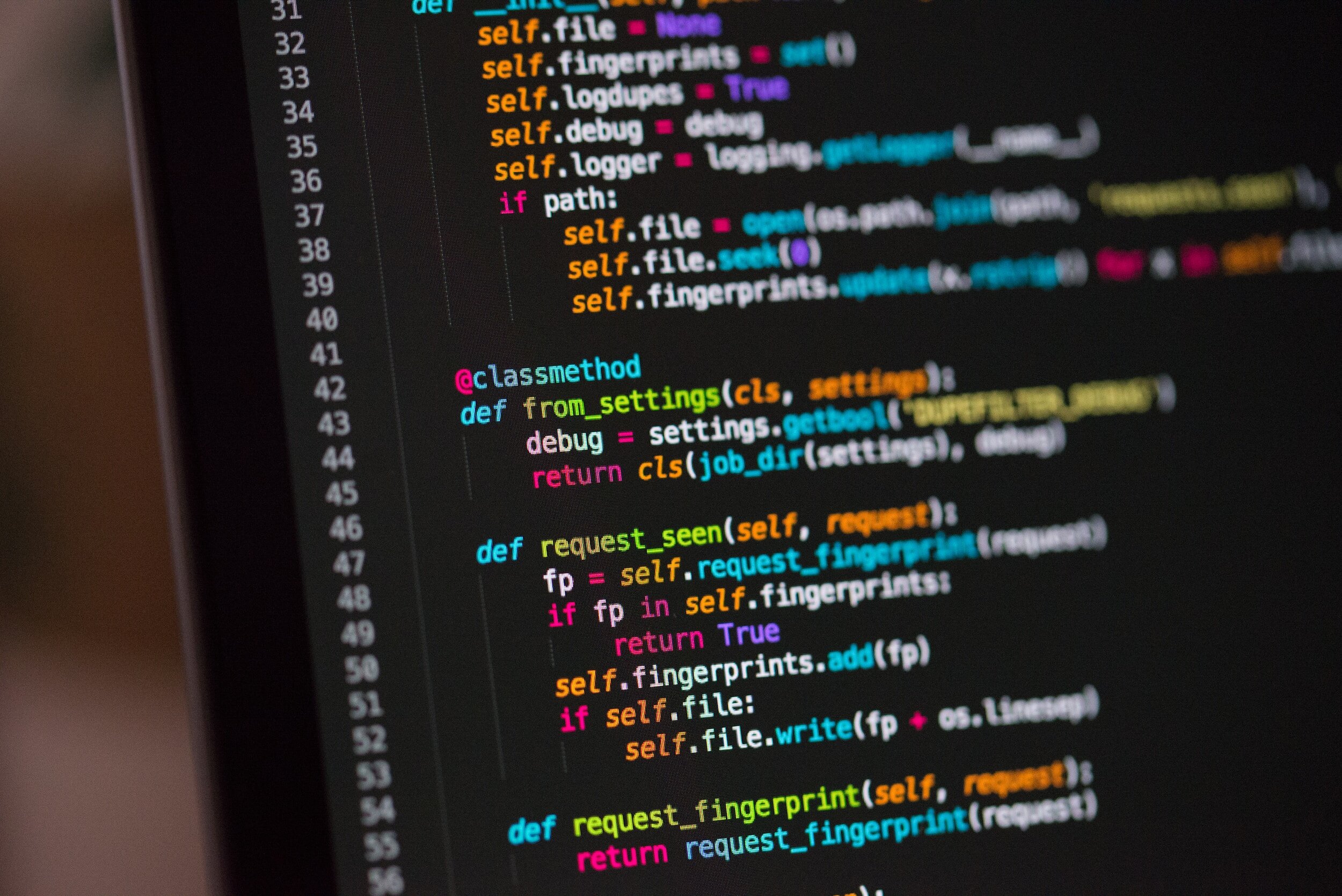
STEM Program
Algorithms, Data Structures and Python
Faculty Advisor: Professor and Director of Undergraduate Studies, Mathematics, Georgia Institute of Technology
Research Practicum Introduction
Algorithms are the heart of computer science. The subject has countless practical applications as well as intellectual depth. In this program, you will learn Algorithms, Data Structures and the programming language Python. Students that enjoy mathematics and/or computer science will enjoy this program. After completing this program, students will understand how many real-world programs work, and will also be able to write their own programs in Python.
Algorithms require data. When we think of data, we think of tables with information, like excel spreadsheets. But data is stored in different forms. These different forms are called data structures. Examples of data structures that you will learn about in this program include queues, trees, stacks. The selection of the right data structure is fundamental to the development of efficient algorithms. Python is one of the most modern and used programming languages.
In this program, you will learn the most powerful and commonly used classes of algorithms, how data is arranged in different data structures to support the algorithms, and the programming language Python to implement the different algorithms. You will be provided with videos and other material to supplement your learning (including programming exercises to practice). Your assignment will be to select a project from the list. This project will lead to your programming an algorithm in Python and writing a paper explaining your work and findings. You will be asked to select your project within two weeks of the first meeting. You will be asked to do a small informal presentation (5 to 10 minutes) during the last meeting and complete your paper.
Possible Topics Covered in Meetings:
Why study algorithms?
Introduction to Python: data types, variables, and basic operations
Basic algorithms and their implementation in Python
Fibonacci numbers: Naïve vs Efficient algorithms
Greatest common divisor and Euclidean algorithm
Big-O notation and computing run times
Greedy algorithms: Main ideas and examples
Dynamic programming: revisiting the money change problem
Python implementations of greedy and dynamic programming algorithms
Divide and conquer algorithms: Naïve sort vs Merge sort
Probability: outcomes, events, and random variables
Simulations in Python
Trees, binary trees, and object-oriented programming in Python
Graphs and graph algorithms
Introduction to video game creation using Pygame
Possible Topics For Final Project:
Design Your Own Video Game with Pygame
Crack the Code: Build a Sudoku Solver Using Backtracking
Map It Out: Find the Fastest Path with Graph Algorithms
Optimize Your Travel: Create an Algorithm for the Best Flight Itineraries
Decode the DNA: Implement Genome Reconstruction Algorithms
Discover DNA Secrets: Build an Algorithm to Find Functional DNA Segments
Encrypt and Secure: Develop a Cryptographic Algorithm
Shape the World: Apply Computational Geometry to Build Maps
Shrink It Down: Master File Compression Algorithms
Balance the Books: Create a Cash Flow Minimizer Algorithm
Conquer Mazes: Design and Code a Maze-Solving Algorithm
Or other topics in this subject area that you are interested in, and that your professor approves after discussing it with you!
Program Detail
Cohort Size: 3-5 students
Duration: 12 weeks
Workload: Around 4 hours per week (including class and homework time)
Target Students: 9-12th grade students interested in Mathematics, Computer Science, Machine Learning, and/or Interdisciplinary STEM studies.
Prerequisites: None. Students are not expected to know Python, to have any programming experience or know anything about Algorithms or Data Structures. The faculty advisor will provide all the necessary materials to learn.
Schedule: TBD (meetings will take place for around one hour per week, with a weekly meeting day and time to be determined a few weeks prior to the class start date)